Jump through / one way platforms
Some adjustments required for GameMaker Studio 2.3
The following article is for pre 2.3 versions of GameMaker Studio 2. It doesn’t take advantage of functions, chained accessors, structs and other new features of GML/GMS2.3+ IDE. Unfortunately I’m unable to update the series for the foreseeable future, but downloading and importing the final project inside the new IDE should trigger the conversion.
It will run correctly. From there you could refactor and clean the code a bit to suit your taste, I guess.
In this article we’ll see how to implement jump through / one way platforms.
If you remember from my previous article, we kept a separate object hierarchy for jump through / one way platforms. That’s because we use them differently in our collision checking; had we made them children of solid objects, we would have had lots of headaches trying to fix their behavior.
As you might already tell from the name, one-way platforms behave like platforms only when hit from above. They don’t even act as obstacles when you travel horizontally so we can absolutely ignore them in the X axis movement part of our code.
The on_jumpthrough script
This script checks if the object is colliding with a one-way platform 1px below its bbox_bottom. This is not enough though, because we must not collide with such platforms whenever we’re 1px (or more) inside them.
This script also take care of very close one-way platforms; the player might be inside one platform and on top of another one: in that case he must stand on the one-way platform anyway and not fall through it.
///@func on_jumpthrough()
///@desc returns the id of the colliding one-way platform or noone if none
var on_platform = collision_rectangle(bbox_left, bbox_bottom + 1, bbox_right, bbox_bottom + 1, oJumpthrough, false, true)
if !on_platform
return noone
var inside_platform = collision_rectangle(bbox_left, bbox_bottom, bbox_right, bbox_bottom, oJumpthrough, false, true)
if !inside_platform
return on_platform
if on_platform == inside_platform
return noone
return on_platform
The clear advantage of keeping all these platforming behaviors separate, is that we can edit our on_ground()
script and reference our newly created one.
///@func on_ground()
///@desc return instance_id of the colliding ground object or noone if not colliding
return on_wall() || on_slope() || on_jumpthrough()
We just add another testing condition to our on_ground
script and from now on, the whole platformer engine will take into account these one-way platforms.
Getting down from one-way platforms
To get the player down from one-way platforms, we modify the jump behavior of the player (which is defined in its ground state)
if keyboard_check_pressed(ord("X"))
{
if keyboard_check(vk_down) && on_jumpthrough()
y++
else
yvel = -3
state_current = playerStateAir
exit
}
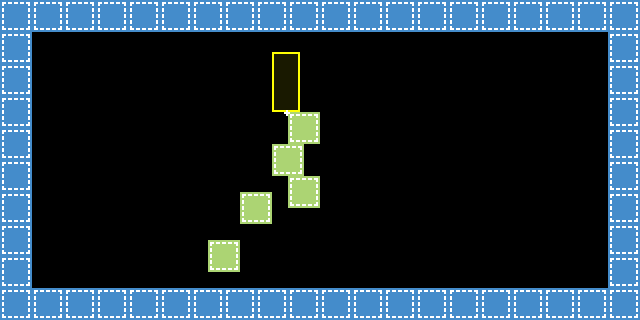
Whenever the player is standing on a jump-through platform and presses jump + the down key, we push the player one pixel inside the platform and then we change its state.
Thanks for the great tutorials! Would it be possible to make jump through/one way slopes with this code? I’ve been tinkering with it but haven’t been able to come up with a solution on my own.
This is great stuff Nick thank you! I am using the most recent version of GameMaker and was encountering some oddities, like not being able to jump. Turns out it was because of the script_execute function in the players step event. It was set to state_current however, there were no instructions for creating a script called “state_current”. After some trial and error I determined that setting this to playerStateAir fixes the issue.